(This article is a work in progress. Keeping it here for now as reference)
This was done using Flutter version 3.27. But will probably work fine with little adjustments in later versions. I’m focusing on Android, but many steps apply for iOS too. I will update the article in the future to cover more platforms.
1. Set Up Firebase Project
- Go to the Firebase Console
- Create a new project or select an existing one.
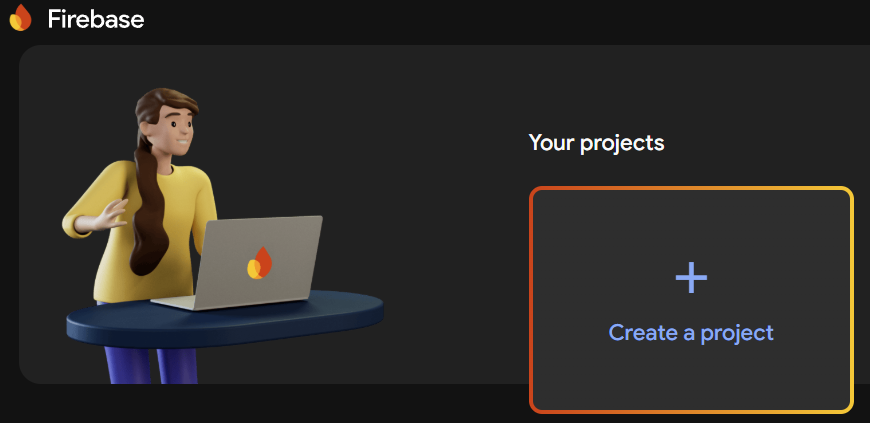
- Add your Flutter app:
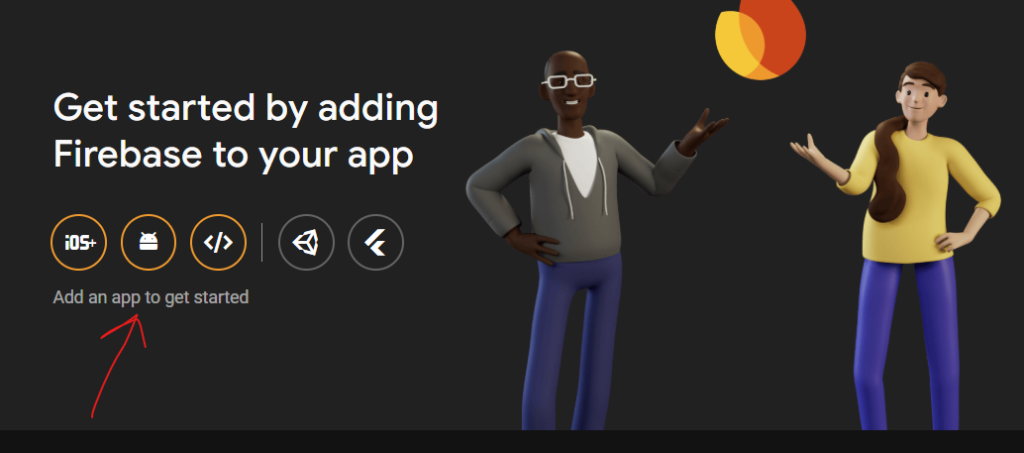
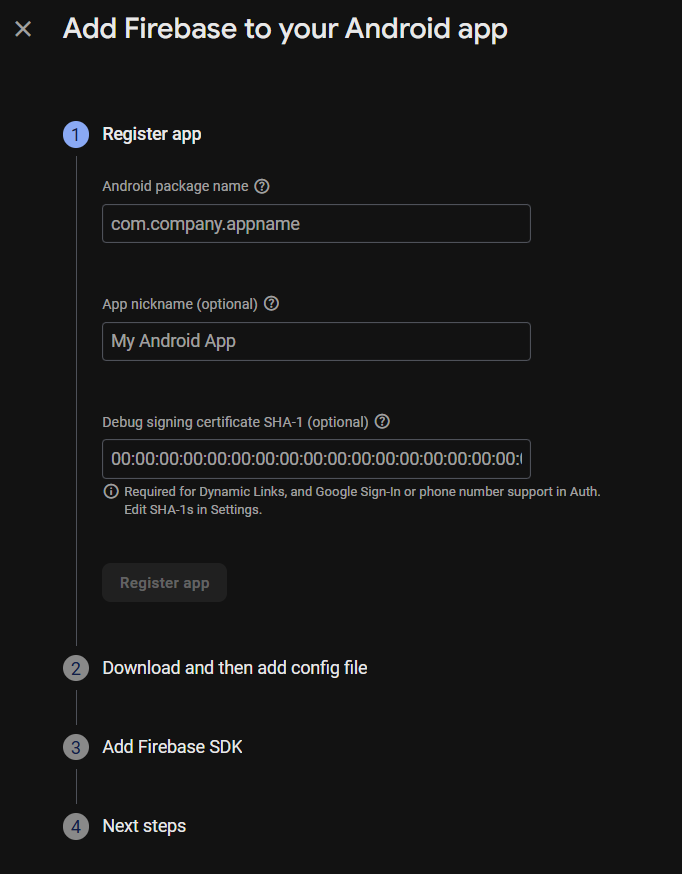
Enter your package name. You can find it in app/build.gradle. Look for applicationId in the defalutConfig.
Then enter a nickname and klick Register App
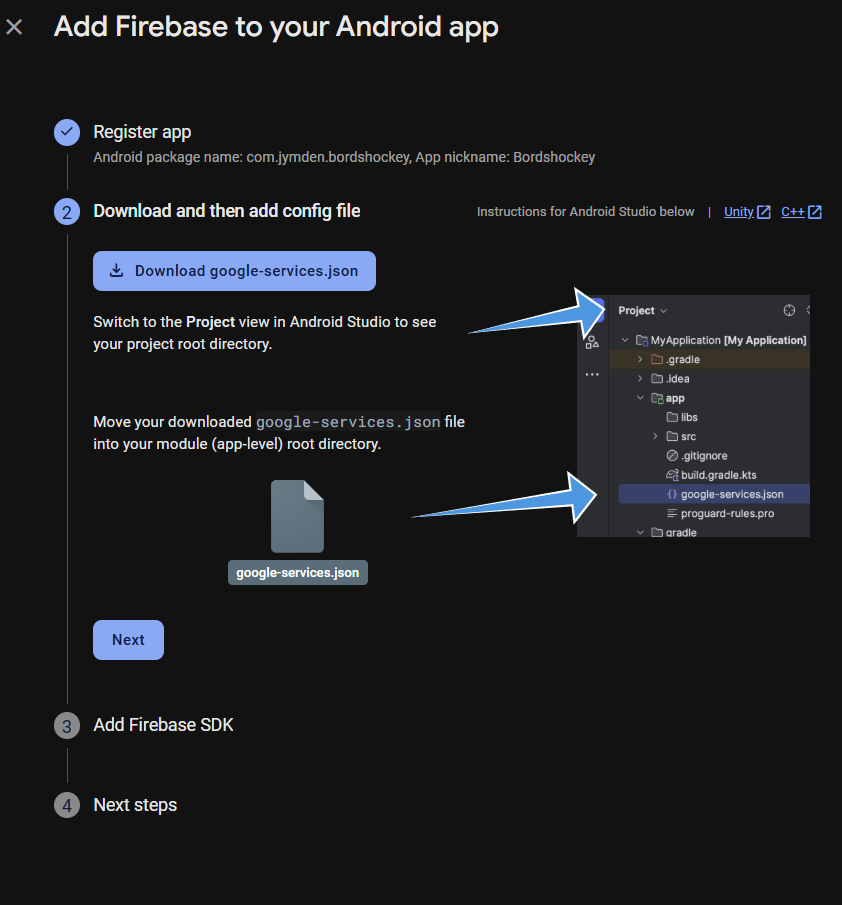
Download the google-services.json
file. and follow the instructions on Firebase.
Put it in the android/app folder.
2. Add Firebase SDK to Your App
- Add the required
firebase_crashlytics
andfirebase_core
dependencies to yourpubspec.yaml
dependencies:
firebase_core: ^3.10.0
firebase_crashlytics: ^4.3.0
YAML- And run
dart pub get
Bash- Add the google services and firebase plugins to Android.
For this you can’t really follow the instructions on Firebase. The documentation for doing Android level stuff in Flutter is sometimes a bit off (even in Flutters own docs).
So if you’re following the guide on Firebase, you’ll probably be looking for the plugins section in your build.gradle. It’s not there… It’s in settings.gradle
Add google services plugin to android/settings.gradle
// android/settings.gradle
plugins {
...
id 'com.google.gms.google-services' version '4.4.2' apply false
id "com.google.firebase.crashlytics" version "2.8.1" apply false
}
KotlinAdd plugins to android/app/build.gradle
// android/app/build.gradle
plugins {
...
id 'com.google.gms.google-services'
id 'com.google.firebase.crashlytics'
}
Kotlin- Install Firebase CLI and FlutterFire
- Use firebase cli to configure:
flutterfire configure
Bash- Select the project you created earlier

- Select the platforms you want to configure, by using the up/down arrow keys and press space to uncheck items. Then press enter.

- Input you package name (the same that you used earlier. That you find in android/app/build.gradle -> applicationId

3. Test Crashlytics
- Enable Crashlytics in Flutter
...
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_crashlytics/firebase_crashlytics.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
// Initialize Firebase
await Firebase.initializeApp();
// Enable Crashlytics
FlutterError.onError = FirebaseCrashlytics.instance.recordFlutterError;
runApp(MyApp());
}
Dart- Throw a test error somewhere in your code. Maybe on a button or gesture detector event. With this simple line:
onTap: () {
FirebaseCrashlytics.instance.crash();
},
Dart- Go to the Firebase console and check for Crashlytics. If it doesn’t show up directly in the left menu, you can go to All Products and scroll down. Under Run, almost at the bottom, you’ll find Crashlytics. Klick it and it will shopw up in the left menu and stay there.
You should have a crash in the list now.
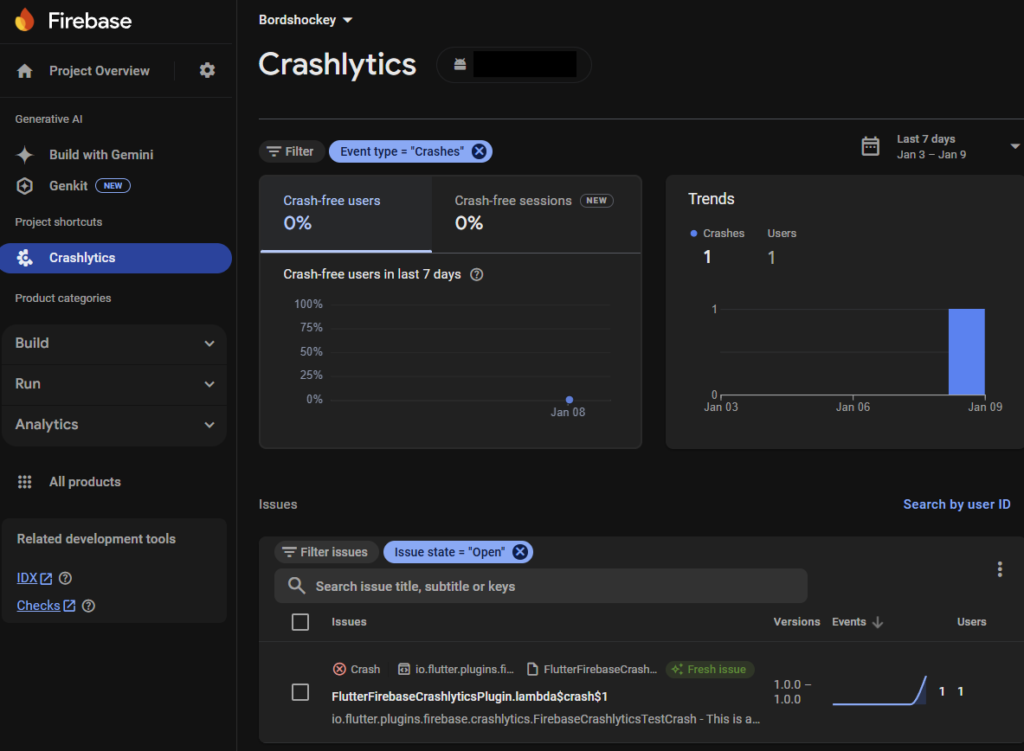
Leave a Reply